

#Python list of dictionaries sum values code
Also note that our code is general: it makes no presumptions about the dictionaries’ keys - they need not be strings nor of any other particular type. This, along with a good docstring, makes our code easy to read, understand, and debug. Note the use of simple and descriptive variables (e.g. we use the variable name key when we iterate over the keys of a dictionary). To get the values that are in the dictionary, we will be using the values() method. The total sum of all the values in the dictionary is printed. The sum() method will return the sum of all values in the dictionary. Parameters - dict1 : Dict dict2 : Dict Returns - Dict The merged dictionary """ merged = dict ( dict1 ) for key in dict2 : if key not in merged or dict2 > merged : merged = dict2 return merged The sum() function is used to find the sum of all the values in the dictionary. We have created a simple and easy function sum() here to add values. Thus calling this function will mutate (change) the state of dict1, as demonstrated here:ĭef simple_merge_max_mappings ( dict1, dict2 ): """ Merges two dictionaries based on the largest value in a given mapping. python Share on : If you want to get all values by using the key name from a list of dictionaries then you can use the below code for that. We can get the sum of values of elements in a dictionary in Python using a for loop. Recall that dictionaries are mutable objects and that the statement merged = dict1 simply assigns a variable that references dict1 rather than creating a new copy of the dictionary. The problem with our function is that we inadvertently merge dict2 into dict1, rather than merging the two dictionaries into a new dictionary. Merged is initialized to have the same mappings as dict1, this is a correct algorithm for merging our two dictionaries based on max-value. The values() method on the dictionary will return a view of the dictionarys values, which can.
#Python list of dictionaries sum values how to
We then set a key-value from dict2 mapping in merged if that key doesn’t exist in merged or if the value is larger than the one stored in existing mapping. Sum Dictionary Values in Python Manav Narula Python Python Dictionary Sum Values in Python Dictionary With the for Loop Sum Values in Python Dictionary With the sum () Function In this tutorial, we will introduce how to sum values in a Python dictionary. Use the sum() function to sum all values in a dictionary.

implementation can make use of Pythons sum function to sum the elements of a list. Thus for key in dict2 loops over every key in dict2. sum a simple numeric list, returns the sums of its items an atom, returns x a list of numeric lists, returns their sums a dictionary with numeric values. The keys and values can be extracted as lists from a dictionary. Let’s discuss different methods to do the task. Recall that iterating over a dictionary will produce each of its keys one-by-one. You have given a list of dictionaries, the task is to return a single dictionary with sum values with the same key. Let’s first see what this function does right.
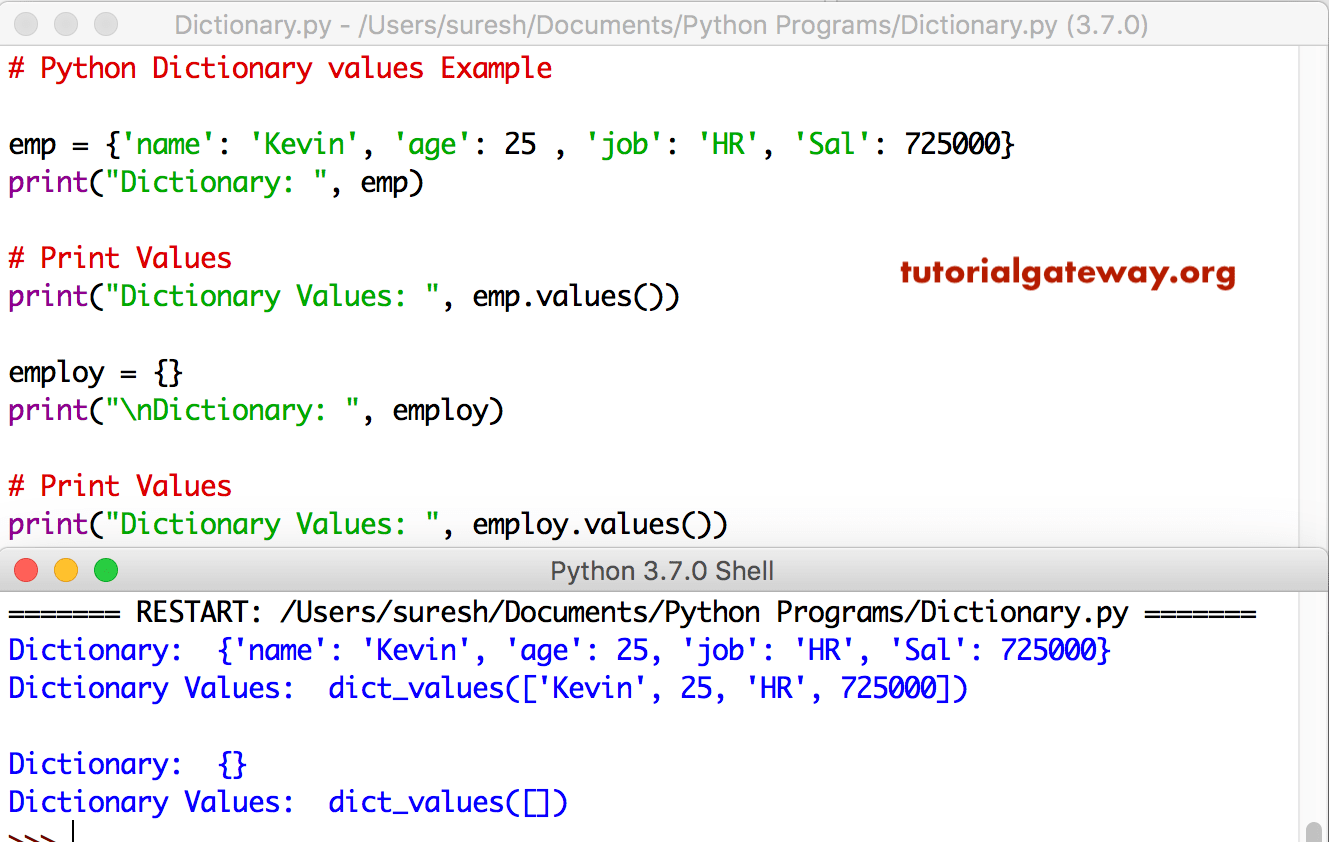
Def buggy_merge_max_mappings ( dict1, dict2 ): # create the output dictionary, which contains all # the mappings from `dict1` merged = dict1 # populate `merged` with the mappings in dict2 if: # - the key doesn't exist in `merged` # - the value in dict2 is larger for key in dict2 : if key not in merged or dict2 > merged : merged = dict2 return merged
